Expandable List View in Android

This is a tutorial based on the Android expandable list view which has the capability to group items and when the user clicks on the group it expands showing items. It can be useful to display a grouped set of items.
ExpandableListAdapter
It loads the data into the items associated with this view.
Some Methods used by this class:-
- (Constructor) ExpandableListAdapter(Context context,List<String> listDataHeader,HashMap<String,List<String>> listHashMap -> Contains context of MainActivity class, List of Headers of each group and list of items of each group.
- getgroupCount() -> returns the size of group list(header list).
- getChildrenCount() -> returns the size of items of group whose groupPosition is given.
- getGroup() -> returns the name of group whose groupPosition is given.
- getChild() -> returns the name of item (whose childPosition is given)of a group( group whose groupPosition is given)
- getGroupId() -> returns the group ID.
- getChildId()-> returns child position.
- isChildSelectable-> returns true as we want child to be selectable.
- getGroupView() -> returns the view for list group header.
- getChildView()-> returns the view for child items.
Some interfaces can be implemented by this class:-
- OnChildClickListener: When a child in the expanded list is clicked, this method is invoked.
- OnGroupClickListener: When a group header in the expanded list is clicked, this method is invoked.
- OnGroupCollapseListener: Used to notify the user when a group is collapsed
- OnGroupExpandListener: Used to notify the user when a group is expanded.
Resource files:-
We have a total of 3 resource files-
- Activity_main.xml– to create a view of the main interface of the application.
- list_group.xml-Layout file to create the header of each group.
- list_item.xml-Layout file to create a list of items of each group.
Read More : Retrieve Image from Firebase Storage in Android
Activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <ExpandableListView android:id="@+id/explv" android:layout_width="match_parent" android:layout_height="wrap_content"> </ExpandableListView> </RelativeLayout>
Create two layout resource files list_group.xml and list_item.xml
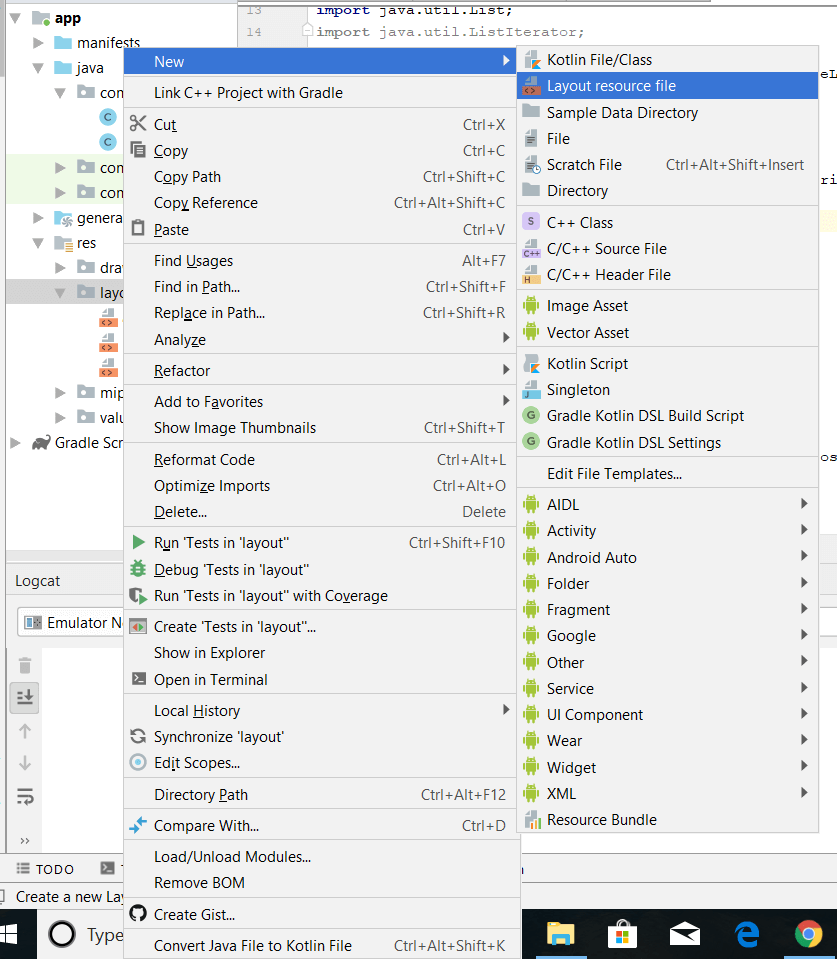
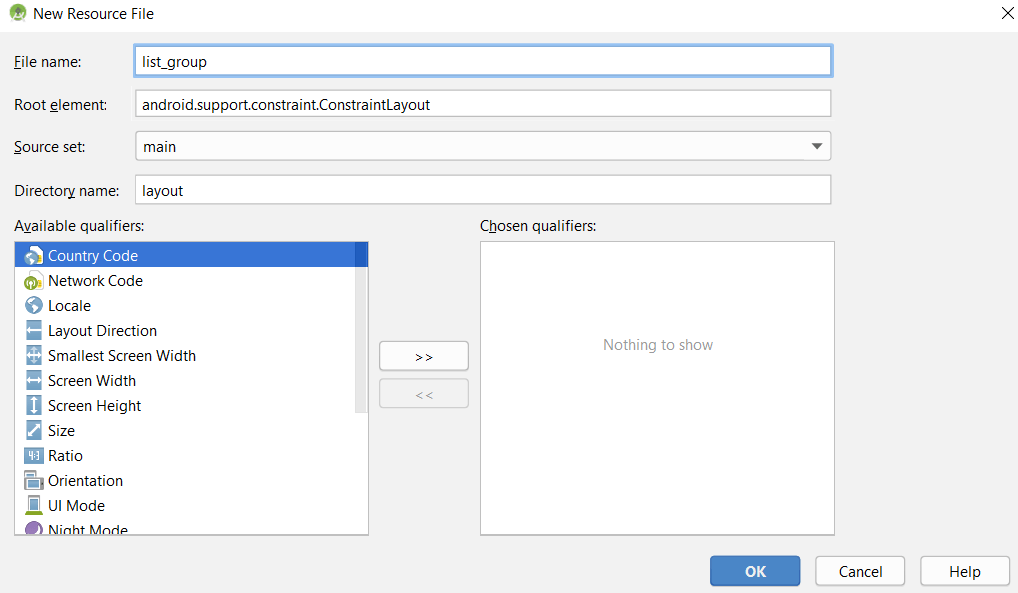
List_group.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/lb1ListHeader" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="16dp" /> </LinearLayout>
List_item.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:padding="8dp" android:background="@color/colorPrimary" android:layout_height="wrap_content" android:orientation="vertical"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/lb1ListItem" android:paddingTop="5dp" android:paddingBottom="5dp" android:textSize="16dp" /> </LinearLayout>
MainActivity.java
package com.example.expandable_listview; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.ExpandableListView; import java.util.ArrayList; import java.util.HashMap; import java.util.List; public class MainActivity extends AppCompatActivity { private ExpandableListView listView; private ExpandableListAdapter listAdapter; //We need a list to create headers/titles of each group and a HashMap to handle item list of each group which is basically a pointer of items to the header of that class. private List<String> listdataheader; private HashMap<String,List<String>> listHashMap; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); listView=findViewById(R.id.explv); initData(); listAdapter=new ExpandableListAdapter(this,listdataheader,listHashMap); listView.setAdapter(listAdapter); } private void initData() { listdataheader=new ArrayList<>(); listHashMap=new HashMap<>(); listdataheader.add("Heading1"); listdataheader.add("Heading2"); listdataheader.add("Heading3"); listdataheader.add("Heading4"); List<String> ed= new ArrayList<>(); ed.add("Example of expandable listview"); List<String> android=new ArrayList<>(); android.add("SubHeading1"); android.add("SubHeading2"); android.add("SubHeading3"); android.add("SubHeading4"); List<String> android1=new ArrayList<>(); android1.add("SubHeading1"); android1.add("SubHeading2"); android1.add("SubHeading3"); android1.add("SubHeading4"); List<String> ed1= new ArrayList<>(); ed1.add("This is expandable listview"); listHashMap.put(listdataheader.get(0),ed); listHashMap.put(listdataheader.get(1),android); listHashMap.put(listdataheader.get(2),android1); listHashMap.put(listdataheader.get(3),ed1); } }
Create another class ExpandableListAdapter.
We can always set items to Expandable ListView using Adapter or List Adapter.
ExpandableListAdapter.java
package com.example.expandable_listview; import android.content.Context; import android.graphics.Typeface; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.BaseExpandableListAdapter; import android.widget.TextView; import java.lang.reflect.Constructor; import java.util.HashMap; import java.util.List; import java.util.ListIterator; public class ExpandableListAdapter extends BaseExpandableListAdapter { private Context context; private List<String> listDataHeader; private HashMap<String,List<String>> listHashMap; public ExpandableListAdapter(Context context,List<String> listDataHeader,HashMap<String,List<String>> listHashMap) { this.context=context; this.listDataHeader=listDataHeader; this.listHashMap=listHashMap; } @Override public int getGroupCount() { return listDataHeader.size(); } @Override public int getChildrenCount(int groupPosition) { return listHashMap.get(listDataHeader.get(groupPosition)).size(); } @Override public Object getGroup(int groupPosition) { return listDataHeader.get(groupPosition); } @Override public Object getChild(int groupPosition, int childPosition) { return listHashMap.get(listDataHeader.get(groupPosition)).get(childPosition); } @Override public long getGroupId(int groupPosition) { return groupPosition; } @Override public long getChildId(int groupPosition, int childPosition) { return childPosition; } @Override public boolean hasStableIds() { return false; } @Override public View getGroupView(int groupPosition, boolean isExpanded, View convertView, ViewGroup parent) { String header=(String)getGroup(groupPosition); if(convertView==null) { //it gives power to embed list_group view into our xml file. LayoutInflater inflater= (LayoutInflater) this.context.getSystemService(Context.LAYOUT_INFLATER_SERVICE); convertView=inflater.inflate(R.layout.list_group,null); } //fetch the parent value and set the value to Textview TextView lbiListHeader= (TextView)convertView.findViewById(R.id.lb1ListHeader); lbiListHeader.setTypeface(null, Typeface.BOLD); lbiListHeader.setText(header); return convertView; } @Override public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertView, ViewGroup parent) { final String childtext=(String)getChild(groupPosition,childPosition); if(convertView==null) { LayoutInflater inflater= (LayoutInflater) this.context.getSystemService(Context.LAYOUT_INFLATER_SERVICE); convertView=inflater.inflate(R.layout.list_item,null); } TextView txtlistchild= (TextView)convertView.findViewById(R.id.lb1ListItem); txtlistchild.setText(childtext); return convertView; } @Override public boolean isChildSelectable(int groupPosition, int childPosition) { return true; } }
After running your application, you will see your interface like this:-
This was a simple tutorial on the Expandable ListView of Android. I hope you like this article. For any Query, comment down below.
STAY TUNED FOR MORE SUCH ANDROID RELATED ARTICLES!!!!!!